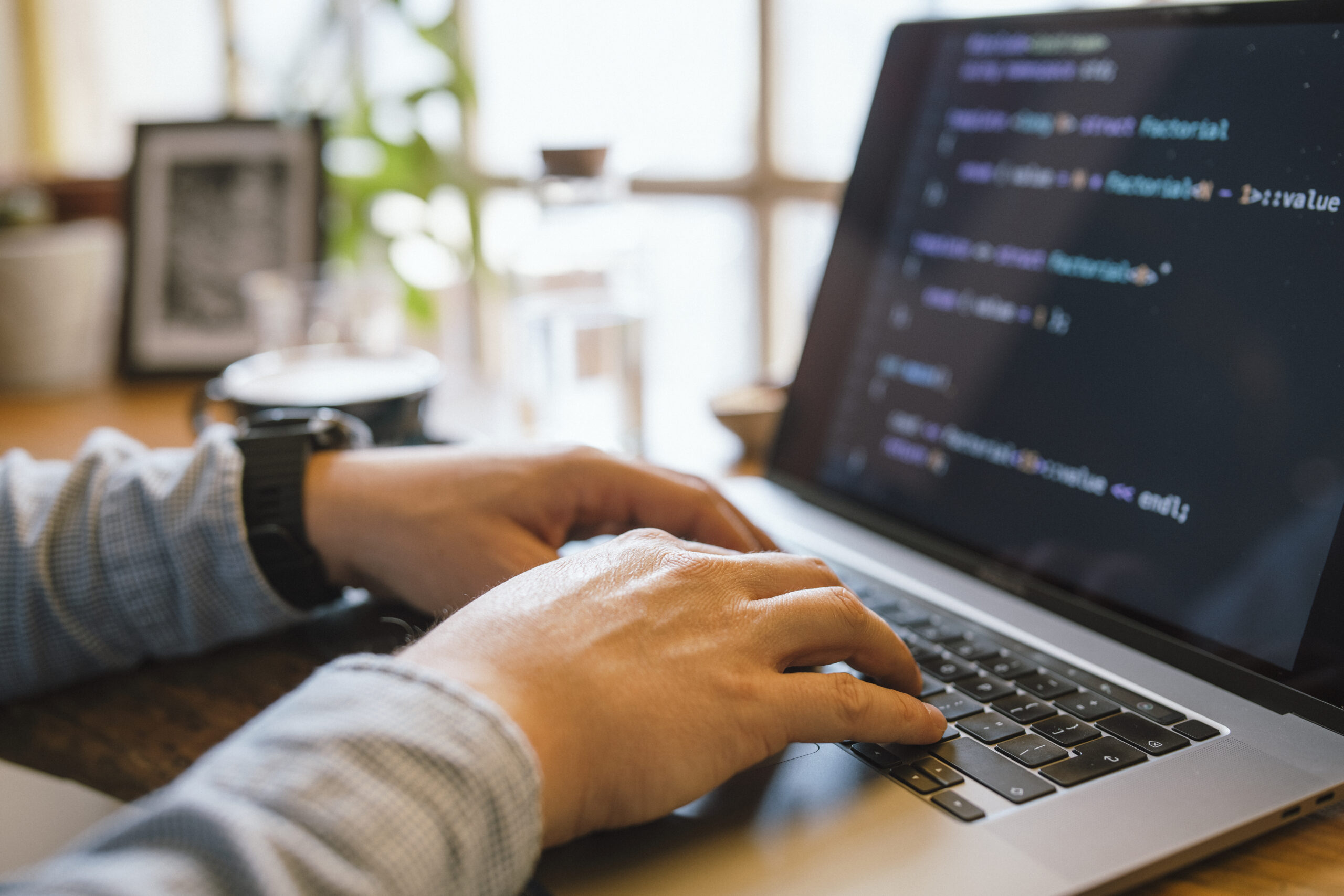
Debugging is One of the more important — nevertheless generally missed — competencies in a developer’s toolkit. It isn't nearly repairing damaged code; it’s about knowledge how and why issues go Improper, and Finding out to Assume methodically to resolve challenges successfully. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of annoyance and considerably transform your efficiency. Here's various tactics to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest methods developers can elevate their debugging competencies is by mastering the instruments they use every single day. Although creating code is one particular Portion of improvement, knowing tips on how to communicate with it successfully in the course of execution is equally significant. Present day improvement environments occur Outfitted with strong debugging capabilities — but lots of developers only scratch the surface of what these applications can do.
Take, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools help you established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code about the fly. When used accurately, they Enable you to notice specifically how your code behaves during execution, that's a must have for tracking down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They let you inspect the DOM, observe network requests, perspective actual-time general performance metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip disheartening UI concerns into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging procedures and memory management. Understanding these tools might have a steeper Finding out curve but pays off when debugging general performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfortable with Variation Command systems like Git to comprehend code historical past, come across the precise instant bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications means going over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress natural environment to make sure that when concerns come up, you’re not misplaced at nighttime. The higher you recognize your equipment, the more time you may expend resolving the particular difficulty as an alternative to fumbling by means of the method.
Reproduce the situation
Among the most important — and sometimes disregarded — measures in efficient debugging is reproducing the problem. Just before jumping into the code or earning guesses, builders want to create a dependable environment or state of affairs wherever the bug reliably seems. Without having reproducibility, fixing a bug results in being a match of probability, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a dilemma is collecting as much context as possible. Check with queries like: What steps brought about the issue? Which ecosystem was it in — enhancement, staging, or creation? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the precise circumstances less than which the bug happens.
Once you’ve gathered enough facts, attempt to recreate the issue in your neighborhood atmosphere. This might mean inputting precisely the same data, simulating very similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the sting circumstances or point out transitions associated. These exams not simply help expose the trouble but will also avert regressions Down the road.
Occasionally, The problem may very well be atmosphere-distinct — it'd come about only on selected operating methods, browsers, or beneath individual configurations. Using resources like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It necessitates patience, observation, along with a methodical strategy. But after you can continually recreate the bug, you might be now midway to fixing it. Using a reproducible circumstance, You should utilize your debugging instruments extra correctly, exam likely fixes safely and securely, and converse far more Obviously using your crew or consumers. It turns an abstract grievance into a concrete challenge — and that’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Improper. As opposed to seeing them as frustrating interruptions, developers should learn to take care of mistake messages as direct communications from the procedure. They frequently show you what precisely took place, in which it happened, and in some cases even why it took place — if you know how to interpret them.
Commence by studying the information meticulously and in comprehensive. A lot of developers, specially when underneath time strain, look at the primary line and right away start building assumptions. But deeper during the error stack or logs might lie the legitimate root bring about. Don’t just copy and paste mistake messages into engines like google — read and fully grasp them very first.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it place to a certain file and line number? What module or purpose triggered it? These inquiries can guide your investigation and level you towards the responsible code.
It’s also helpful to grasp the terminology of the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Studying to acknowledge these can dramatically hasten your debugging method.
Some glitches are imprecise or generic, and in People conditions, it’s essential to look at the context in which the error transpired. Test related log entries, input values, and recent improvements inside the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger troubles and supply hints about probable bugs.
Ultimately, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, aiding you pinpoint troubles more rapidly, lower debugging time, and turn into a extra efficient and assured developer.
Use Logging Properly
Logging is Among the most impressive tools in a developer’s debugging toolkit. When used successfully, it provides true-time insights into how an application behaves, aiding you recognize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
A great logging approach commences with being aware of what to log and at what degree. Frequent logging ranges consist of DEBUG, INFO, Alert, Mistake, and Lethal. Use DEBUG for specific diagnostic facts through growth, Data for common occasions (like successful get started-ups), Alert for opportunity challenges that don’t split the application, Mistake for genuine troubles, and FATAL in the event the system can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure critical messages and slow down your procedure. Center on critical activities, state improvements, input/output values, and important determination points as part of your code.
Format your log messages Evidently and continuously. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even simpler to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in output environments in which stepping as a result of code isn’t feasible.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. Which has a effectively-considered-out logging approach, it is possible to lessen the time it takes to spot difficulties, gain deeper visibility into your purposes, and improve the All round maintainability and trustworthiness within your code.
Believe Just like a Detective
Debugging is not simply a technological job—it is a method of investigation. To efficiently discover and take care of bugs, builders should strategy the method similar to a detective resolving a mystery. This attitude can help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys a criminal offense scene, collect as much relevant information as you are able to with out jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a clear picture of what’s happening.
Next, variety hypotheses. Talk to you: What can be resulting in this habits? Have any alterations a short while ago been built to your codebase? Has this situation transpired prior to under identical situation? The purpose is always to slim down choices and identify probable culprits.
Then, examination your theories systematically. Make an effort to recreate the problem inside of a controlled atmosphere. If you suspect a certain purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Spend close focus to small information. Bugs frequently disguise inside the the very least anticipated places—just like a missing semicolon, an off-by-a person error, or simply a race issue. Be thorough and individual, resisting the urge to patch The difficulty with no fully comprehension it. Temporary fixes may conceal the actual issue, just for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and assistance Other people fully grasp your reasoning.
By thinking like a detective, builders can sharpen their analytical competencies, approach issues methodically, and develop into more effective at uncovering hidden concerns in advanced units.
Create Exams
Producing checks is among the most effective solutions to improve your debugging abilities and Total progress performance. Checks not only assist catch bugs early but additionally serve as a safety Web that offers you assurance when making modifications for your codebase. A effectively-examined application is easier to debug since it permits you to pinpoint specifically where by and when a dilemma takes place.
Get started with device assessments, which target specific features or modules. These modest, isolated exams can swiftly reveal irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a check fails, you immediately know where to look, significantly lessening some time invested debugging. Unit checks are In particular useful for catching regression bugs—problems that reappear following previously being mounted.
Following, integrate integration exams and finish-to-end checks into your workflow. These support make sure several areas of your software get the job done collectively smoothly. They’re significantly useful for catching bugs that come about in sophisticated systems with several factors or providers interacting. If some thing breaks, your checks can let you know which Element of the pipeline failed and underneath what conditions.
Creating checks also forces you to Imagine critically about your code. To check a characteristic properly, you may need to know its inputs, predicted outputs, and edge instances. This degree of being familiar with By natural means potential customers to better code framework and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the take a look at fails persistently, you can give attention to correcting the bug and watch your examination go when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Later on.
Briefly, writing exams turns debugging from the frustrating guessing sport into a structured and predictable course of action—helping you catch a lot more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Using breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
When you are far too near to the code for far too very long, cognitive tiredness sets in. You would possibly start overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a espresso break, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Numerous developers report getting the basis of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially all through extended debugging periods. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping away enables you to return with renewed Electrical power and also a clearer attitude. You might out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular below limited deadlines, however it actually contributes to faster and simpler debugging Ultimately.
In brief, getting breaks isn't a sign of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your viewpoint, and allows you avoid the tunnel eyesight That always blocks your development. Debugging is actually a psychological puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each and every bug you face is a lot more than simply a temporary setback—It really is a chance to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, each can instruct you something beneficial in case you make the effort to replicate and review what went Incorrect.
Commence by asking by yourself some important concerns after the bug is settled: What induced it? Why did it go unnoticed? Could it are already caught before with superior techniques like device tests, code opinions, or logging? The responses generally expose blind places with your workflow or knowledge and make it easier to build much better coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. Eventually, you’ll begin to see designs—recurring concerns or frequent problems—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug along with your peers is often Specially effective. Whether or not it’s via a Slack concept, a short publish-up, or a quick awareness-sharing session, supporting Many others stay away from the exact same difficulty boosts crew effectiveness and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a more info few of the greatest builders usually are not those who create great code, but people who consistently discover from their issues.
Ultimately, Just about every bug you repair provides a new layer in your talent set. So up coming time you squash a bug, have a moment to mirror—you’ll appear away a smarter, far more able developer due to it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at That which you do.